RoboBoard X4
Motor driver firmware
New driver firmware v1.60 is available. See Release notes and Driver update
A more powerful programmable controller, designed for medium to larger sized robots with high voltage motors and additional expansion options.
This compact package features components that are essential for robotic applications: battery input, charging, motor drivers, modules and sensors extensions, orientation sensor, powerful processor, wireless connectivity, software support and more.
To start programming install Arduino and check RoboBoard API section.
Find at: Totemmaker.net store → RoboBoard X4
Details
Click orange box to jump to explanation
Processor:
• ESP32 module (ESP32-D0WD)
• Dual-core 240Mhz (Xtensa LX6)
• 320KB SRAM, 8MB flash
• Bluetooth (classic and BLE)
• Wi-Fi
Board features:
• 3 Servo motor ports (5 Volt)
• 4 DC motor ports (11.1 Volt)
• 4 GPIO pins
• 4 RGB lights
• IMU sensor (accel and gyro)
• Reset, User buttons
• Status LED
• On/Off switch
• DC power input
• Battery input, integrated charger
• Qwiic port (STEMMA QT compatible)
• TBUS (CAN bus)
• USB (data only, no power)
Power:
• DC adapter: 30W, 15V, 2A
• Battery: 3S Li-Ion 11.1V 2200mAh
Dimensions:
• 70 x 50 x 14 mm (L x W x H)
ESP32
RoboBoard is powered by ESP32 - a capable SoC with rich peripherals and wireless connectivity. This combinations makes it perfect for robotic applications where motor driving and wireless control is required.
For a past few years ESP32 is one of the most popular microcontroller among maker community. While its hardware capabilities are no doubt it also comes with vast software support, maintained officially by Espressif company and a help from community. This led to well established Arduino support for ESP32, powering many projects all over the world. Our Arduino support for RoboBoard is based on official core for maintaining compatibility with libraries and examples with addition to well integrated RoboBoard features.
For more ESP32 specific details read ESP32 section.
Board features
Qwiic port
Wires:
• Black = GND
• Red = 3.3V
• Blue = SDA
• Yellow = SCL
Cable: standard "Qwiic cable". Can be found in local electronics store.
Connector: SM04B-SRSS-TB 4-pin
A connection system to attach third-party I2C modules. This allows to choose from many available sensors and other interface devices. Small and sturdy connector eliminates need for soldering and enables plug-and-play style modular systems. Each module comes with its own Arduino library (supplied by manufacturer).
This port is compatible with SparkFun Qwiic and Adafruit STEMMA QT modules.
For using Qwiic modules with RoboBoard read GPIO / Qwiic section.
TBUS (CAN bus)
Wires: CAN-H, CAN-L, GND, 5V
(out), BATT
(~12V), N.C. (pinout)
Cable: Totemmaker.net store → TOTEMBUS CABLE
Connector: Micro-MaTch 6-pin
TBUS (TotemBUS) connectors with dual purpose: Plug into CAN bus networks or attach X4 extension modules. Both are interconnected and used for daisy-chaining.
For more information read CAN section.
void setup() {
// Start CAN peripheral at 500kbps
CAN.begin(500);
}
void loop() {
// Wait for CAN packet receive. 300ms timeout
if (CAN.readPacketWait(300)) {
// Get received packet
auto packet = CAN.getPacket();
// packet.id, packet.data, packet.len, packet.ext, packet.rtr
}
// Send CAN packets
uint8_t data[8] = {1,2,3,4,5,6,7,8};
CAN.writePacketExt(0x112233, data, 8); // Extended
CAN.writePacketStd(0x1AB, data, 8); // Standard
}
USB port
USB connector is present for Serial Monitor and Arduino firmware upload.
Board cannot be powered from USB alone! It requires either Power or Battery input.
Serial converter: CP2102N
Connector: miniUSB
IMU sensor
6DOF IMU sensor (3-axis accelerometer and 3-axis gyroscope) allows to detect board movement and orientation. Very useful in many robotic projects. Chip is connected to I2C line (same as Qwiic connector) and can be accessed trough API functions IMU
.
#include <Wire.h>
void setup() {
Serial.begin(115200);
Wire.begin();
}
void loop() {
auto result = IMU.read(); // Read sensor measurements
Serial.println(result.getX_G()); // Read unit from "result"
delay(100); // Wait 100ms for next read
}
Status LED
Red LED next to user button for indicating board state:
Short rapid blink - board started.
Blink 3 times - battery is depleted.
Blinking constantly - driver initialization error (consult forum).
On - running.
This LED can be controlled from Arduino code:
LED.on(); // Turn LED On
LED.off(); // Turn LED Off
LED
section.
RGB lights
Back side of the board contains 4 RGB lights for using with multiple purposes.
Note: some features must be enabled in Board settings.
Connection indication (Totem App):
- Running animation - no connection
- Steady color - connected to robot
Appearance customization (Totem App):
- Change color - click Settings (when connected) and drag slider to configure robot RGB and connection color.
Battery State Of Charge:
Upon power on - battery charge level will be displayed by playing "loading" animation with specific color:
- - battery is full
- - battery is medium
- - battery is low
- - battery is discharged (blink 3 times)
In case battery is too low - board will power itself off, indicating by Status LED.
Programming:
Use RGB
API to change colors to your likeness or application.
RGB.color(Color::Green); // Color name
RGB.color(0, 0, 125); // RGB value
Buttons
Contains two types of buttons:
RESET
- Performs hardware processor reset. Used to restart currently running program.BUTTON
- Programmable user button. Can be used withButton
API.
By default BUTTON
is inactive and left for user implementation.
void setup() { }
void loop() {
if (Button.wasPressed()) {
LED.off();
}
else if (Button.wasReleased()) {
LED.on();
}
}
GPIO pins
Pins for external IO and communications (UART, I2C, SPI, ...):
- VCC - 3.3 Volt output pin.
- GPIOA - GPIO pin
14
- GPIOB - GPIO pin
23
- GPIOC - GPIO pin
25
- GPIOD - GPIO pin
26
- GND - Ground pin
Connector: 0.1″ (2.54 mm) female pin header
void setup() {
pinMode(GPIOA, OUTPUT); // Configure pin to output
pinMode(GPIOB, INPUT); // Configure pin to input
digitalWrite(GPIOA, HIGH); // Set pin state
int state = digitalRead(GPIOB); // Read pin state
}
void loop() { }
For more information read GPIO / Qwiic section.
Motor drivers
Integrated drivers allows to connect motors directly to the board, eliminating the need for an external modules. All control functions are built into RoboBoard API DC
, Servo
and includes some more advanced features:
DC
- control % of power, electric braking, audible tone generation, acceleration and deceleration control, power limiter, configurable frequency, spin direction invert.Servo
- position, angle, pulse control, speed control, timed sequences, configurable pulse range, configurable period, trimming, spin direction invert.
Servo motor ports
Servo wire colors:
• (-) Brown = GND
• (+) Red = VCC
• (S) Orange = Signal (PWM)
Connector: 0.1″ (2.54 mm) male pin header
Individual channels for connecting standard (3 wire) servo motors and other electronics. Pins are marked with letters A, B, C for controlling up to 3 motors with regulated 5 Volt output.
By default, API is configured for 180 degree servo motors, with pulse duration between 500μs-2500μs and period of 20ms (50Hz). These parameters can be customized.
For more information read Servo
section.
DC motor ports
Connector: JST-PH 2-pin
Connectors for 12V brushed DC motors. Ports are marked with letters A, B, C, D for controlling up to 4 motors.
Power comes straight from the battery (trough H-bridge motor driver) and peak voltage is dependent on State Of Charge (8.4-12.6V).
Motor power is controller using 20kHz PWM signal (driver v1.60 required). This parameter can be customized.
Board contains dual DRV8847S driver chips for total control of 4 individual motor ports.
Each driver is rated for 2 Amp peak and grouped into AB and CD ports. In case motor consumes too much current - a thermal protection will trigger when IC heats up over 150°C. After cool down - it turns back on. This may cause motor power off once a second.
Motors shipped with Totem kits consumes 1.5A at stall and does not trigger thermal protection (only case if motor is stalled for a long time).
For more information read DC
section.
Note: motor wire colors (red, black) does not indicate polarity (+, -). Swapping wires only changes spin direction.
Power circuit
Board contains built-in electronics for power distribution and control. It takes care of battery charging and provides power for all the components:
BATT
(6 Amps) - used byDC motor ports
,CAN bus BATT pin
. Vary between 8.4-15 V5V
(6 Amps) - used byServo motor +
,RGB lights
,CAN bus 5V pin
3.3V
(2 Amps) - used byESP32
,co-processor
,IMU
,GPIO VCC
,Qwiic port
USB port is for data only and does not power 5V
or 3.3V
rails. Either battery or DC adapter is required to program and power up the board.
Charging
Battery charging circuit activates once both battery and DC adapter are plugged in and continues until battery is full. Charging process indicated by status LED (near to DC jack):
Blinking - battery not detected .
On - battery is charging .
Off - battery is charged .
Note: Sometimes LED can start blinking when battery is charged.
Power input
DC barrel-jack input to supply power for board and and battery charging. When connected it starts charging the battery (if not full) and powers BATT
rail (overrides battery). In this case BATT
will become 15V and DC motors will spin a little faster. If board starts to consume more than 2 Amps, missing energy will be topped off from the battery.
Input voltage: 15 Volts
Connector: barrel jack 5.5/2.0mm center-positive
Recommended to use only supplied DC power adapter: 30W, 15V, 2A power adapter
Battery input
Battery input for board power and charging. When connected it powers BATT
rail. Voltage may vary between 8.4-12.6 V, depending on battery State Of Charge.
Input voltage: 8.4-12.6 Volts
Battery type: 3S 11.1V Lithium (rechargeable). Under-voltage protection required
Connector: JST-VH 2-pin
Important notices:
- Does not feature low voltage protection!
Battery must contain its own under voltage protection circuit. - Does not feature reverse voltage protection!
Make sure polarity ( + - ) is correct before plugging battery in. - Do not connect other power sources (or different battery types)!
May be damaged when DC power jack is plugged in and charging starts.
Recommended to use only supplied battery: 3S Li-Ion, 11.1V, 2200mAh battery
On/Off switch
Used to turn X4 board power on/off without disconnecting DC jack or battery.
Upon power on - battery charge level (if enabled) will be displayed. "Loading" animation will be played with specific color:
- - battery is full
- - battery is medium
- - battery is low
- - battery is discharged (blink 3 times)
Setting to OFF position shuts down all power rails (BATT
, 5V
, 3.3V
).
Battery can still be charged while switch is set to OFF position.
Driver update
RoboBoard contains additional processor for low-level control operations (motor, board, RGB). It works by communicating over UART and relieving some strain from ESP32. This processor is called "motor driver" and runs its own firmware.
View installed version:
Run this Arduino code and open Serial Monitor. Latest version is mentioned in Releases.
void setup() {
Serial.begin(115200); // Setting baud to 115200
}
void loop() {
Serial.print("Driver: ");
Serial.println(Board.getDriverVersionStr());
delay(500);
}
Update to latest firmware:
- Use Arduino IDE and install latest Totem Boards.
- Power on RoboBoard X4 and connect to PC.
- Select
Tools
→Board
→Totem Boards
→RoboBoard X4
.
SelectTools
→Port
.
SelectTools
→Programmer
→Esptool
. - Click
Tools
→Burn bootloader
. - Update procedure will start. Wait for (red) LED to light up and RGB lights go green.
Troubleshooting:
If (green) RGB is blinking - firmware is already flashed.
If (red) LED starts blinking - there was update error. Try press RESET button and wait.
If (red) LED still blinks, follow these steps:
- Open
Tools
→Serial Monitor
. - Select
115200 baud
. - Wait for Serial output. Error will be displayed. Contact support for further help.
Schematics
Mechanical drawing (dimensions):
Revision changelog
We are always looking to improve our products. Any physical change (components, layout) is indicated with board revision number (printed on top). Each revision may have different features or functionality.
v1.1
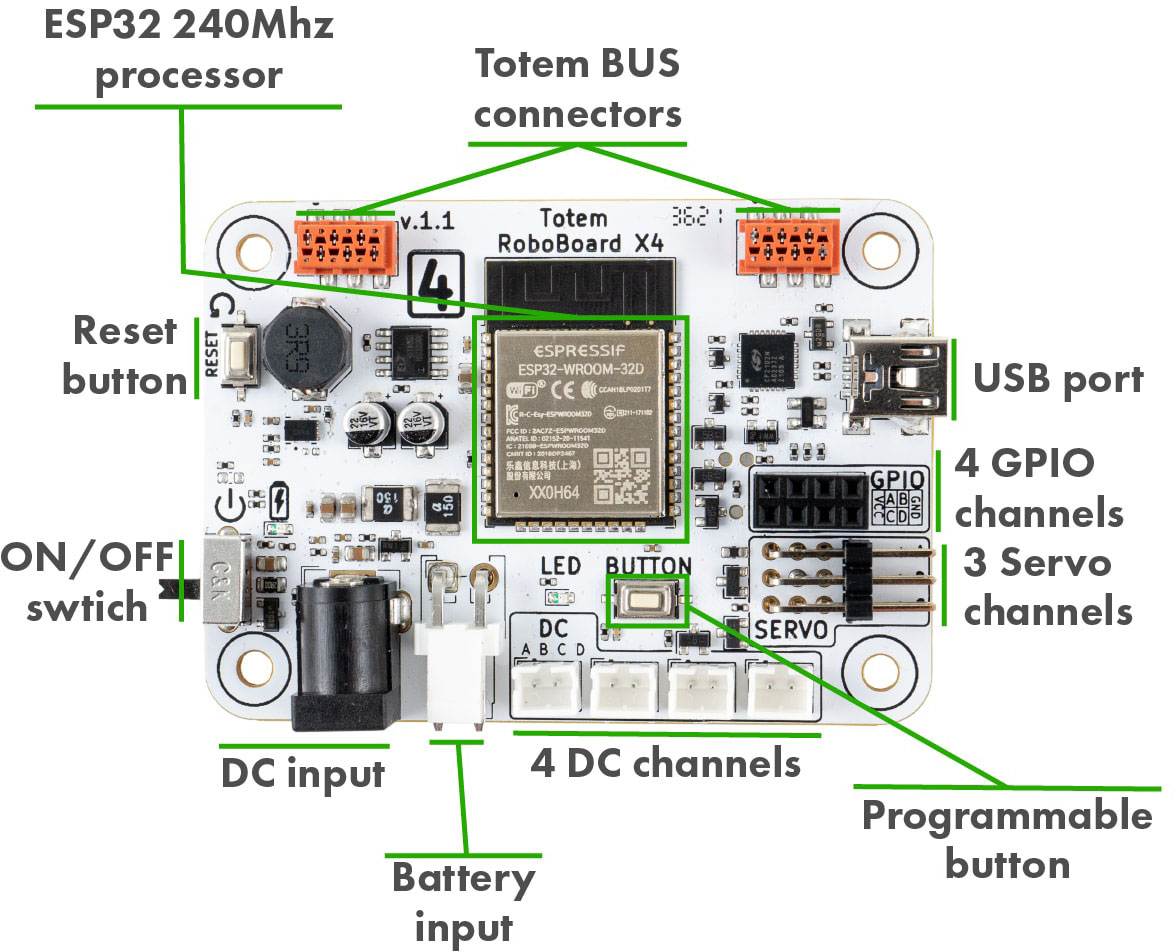
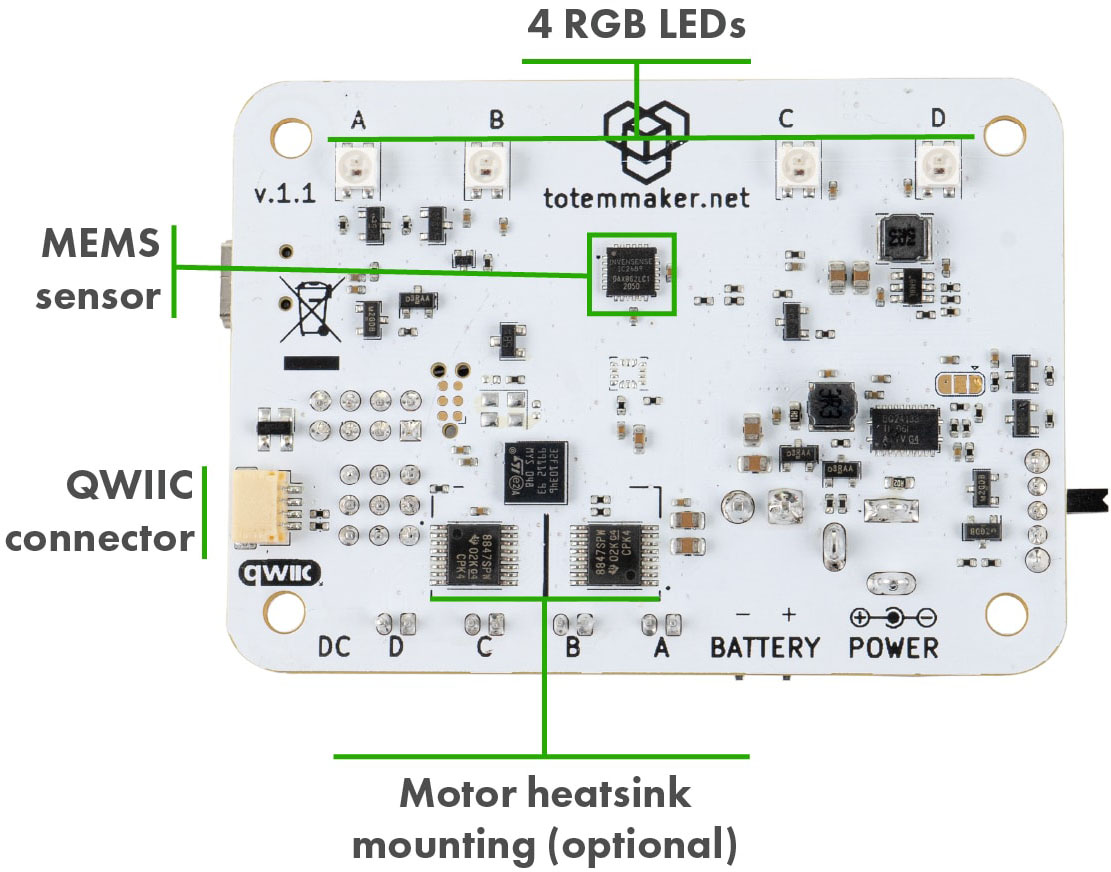
- Connected GPIO pins to ESP32
- Switched to addressable RGB lights
- Added Reset button
- Added Qwiic port
- Changed IMU to ICM-20689
v1.0
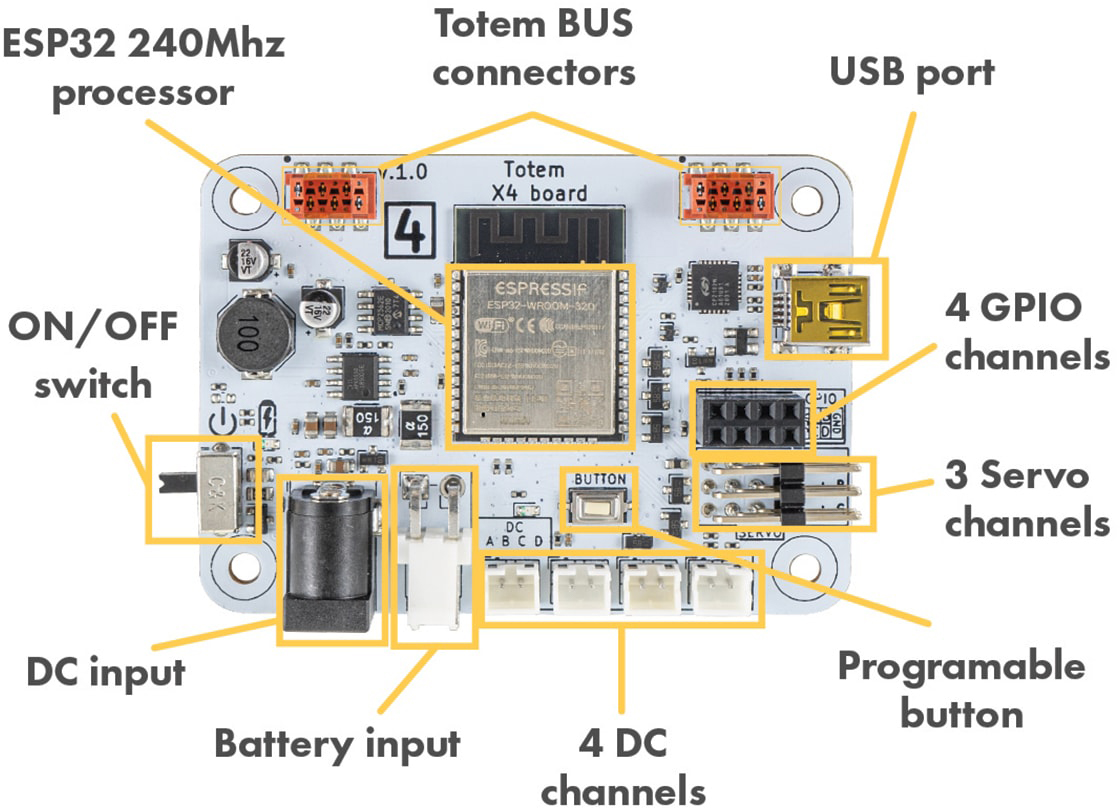
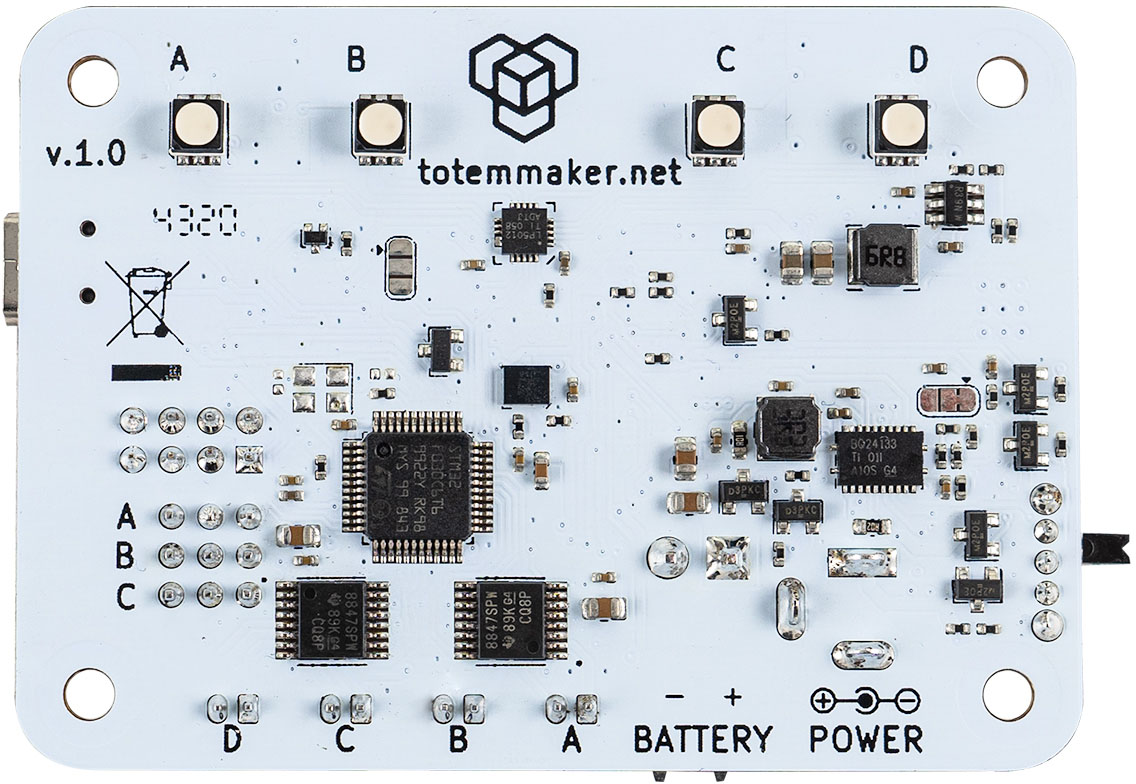
- Initial release